Lazy load images sooner when idle
I was working on a mini cart experience for an e-commerce store. A mini cart provides a preview of the items in your cart, usually with small thumbnails of the products.
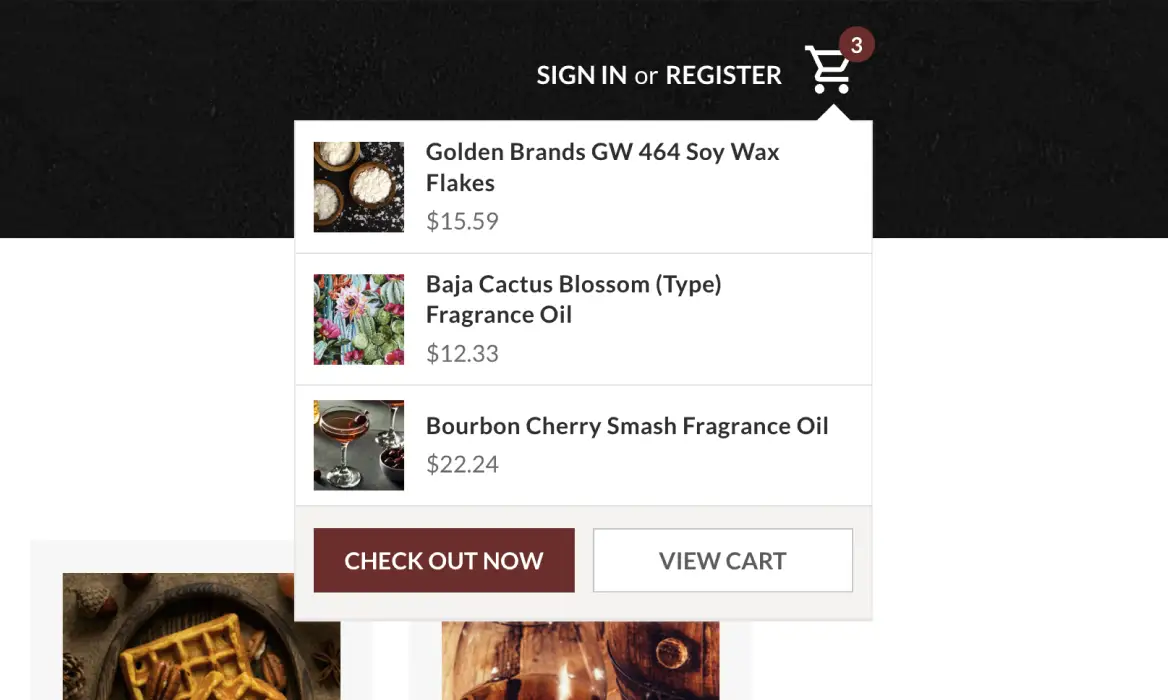
I initially added loading="lazy"
to these images since they aren't critical and are at the top of the document, potentially loading items not needed until later. However, when opening the mini cart, the images would load after the mini cart flyout appeared, making the site feel slower, even though the page had finished loading some time ago.
I discovered a little trick to preload these images by swapping loading="lazy"
with loading="auto"
after the whole page was finished loading. This essentially creates a progressive preload; the images will load if they are in view or when there is nothing else left to load.
The script
<img src="photo.jpg" loading="lazy" data-idleload>
<script>
// IdleLoad.js
// Swap loading=lazy to loading=auto after the whole page is loaded
function idleLoadImgs() {
var idleloadImages = document.querySelectorAll('[data-idleload]');
idleloadImages.forEach(img =>
img.setAttribute('loading', 'auto')
);
};
window.addEventListener('load', idleLoadImgs);
</script>